STM32 Software SPI SSD1331 Sketch
In a previous post, I wrote about designing a ‘breakout board’ for an SSD1331
OLED display with 96×64 pixels and 16 bits of color per pixel. With the hardware already put together, this post will cover writing a basic software driver for the displays. To keep things simple, we will talk to the display using software SPI functions instead of the STM32’s SPI hardware peripheral.
If you want to skip assembling your own boards, you can also buy a pre-made display such as this one sold by Adafruit. They have also written a library for these displays which works with several common types of microcontrollers, if you just want to use them without worrying about the display settings. But if you want to try understanding this sort of communication at a lower level, read on!
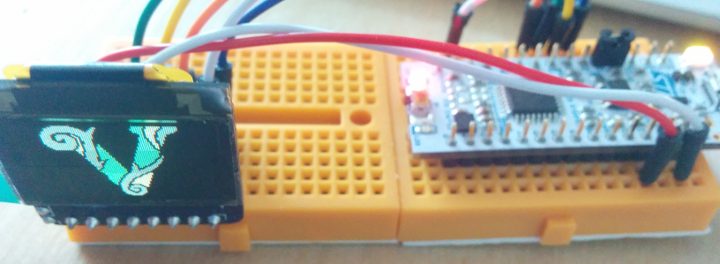
The finished program will display a predefined framebuffer, like this little logo!
Since many small microcontrollers – including the STM32F031K6
discussed in this example – don’t have 12KB of RAM available to store a 96×64 display at 16 bits per pixel, I’ll use a framebuffer with just 4 bits per pixel in this example (3KB), and map those 16 values to a palette. This example builds on the first few “Bare Metal STM32 Programming” tutorials that I’ve been writing, so here is a Github repository with the entire example project (including supporting files) if you don’t want to read those.
DIY OLED Display Boards: SSD1306 and SSD1331
OLED displays are excellent solutions for low-power, high-visibility UIs that don’t need to depict much detail and can be smaller than a square-inch or two. These days, they are cheap and available enough to be viable options for the hobbyist:
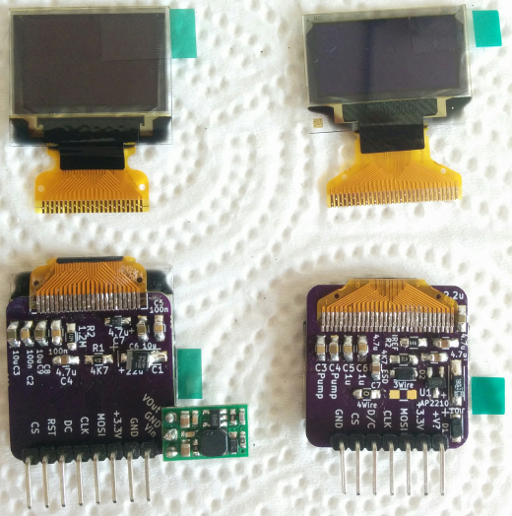
Top: The display panels as they arrive in the mail. Bottom: Boards with the circuits described in this post – the panels are glued to the other side.
These are two small display panels which you can find on Taobao, Alibaba, or eBay in small quantities for roughly $2-4 each. The one on the left is a 96×64-pixel SSD1331
16-bit color display. The one on the right is a 128×64-pixel SSD1306
monochrome display where each pixel is either ‘off’ or ‘on’ – typically ‘on’ is a white or blue color. Some of them have a row of 16px along the top set to yellow, but each pixel is still only one color.
In this post, we will walk through the circuitry (although not the code) required to control these displays using a microcontroller, including circuit schematics for laying out a ‘breakout board’ in your preferred EDA program – I used KiCAD, and I’ll also provide a link to those projects if you don’t want to design a new board.
Writing a Box: SVG Basics
Lately, I’ve been looking for ways to get people interested in basic electronics, and things like kits and/or lessons seem like a great way to do that. I’ve also been looking for ways to learn about making things with a laser cutter, so I decided to put together a stack-able box that could double as storage and a display for available electronics parts at a local makerspace.
The basic idea was simple; start with a box ‘outline’ similar to those generated by Makercase, and then lay out a grid pattern of ‘dotted lines’ to slot crenellated dividers into. I wanted something like a shallow tray, with several horizontal and vertical dividers to hold different types of parts. What could possibly go wrong?
At this point, with a basic idea and maybe a quick sketch, most sane people would open up a program like Autodesk Inventor, Solidworks, or Adobe Illustrator. Options like Inkscape, OpenSCAD, or SolveSpace would also work well if you like free software. But after having some trouble with SVG exports, I wondered if it might make sense to just write the files by hand, for a simple grid pattern.
I guess it depends on your definition of ‘sense’! In this post, we’ll learn how to write SVG files for a small laser-cut ‘test’ box:
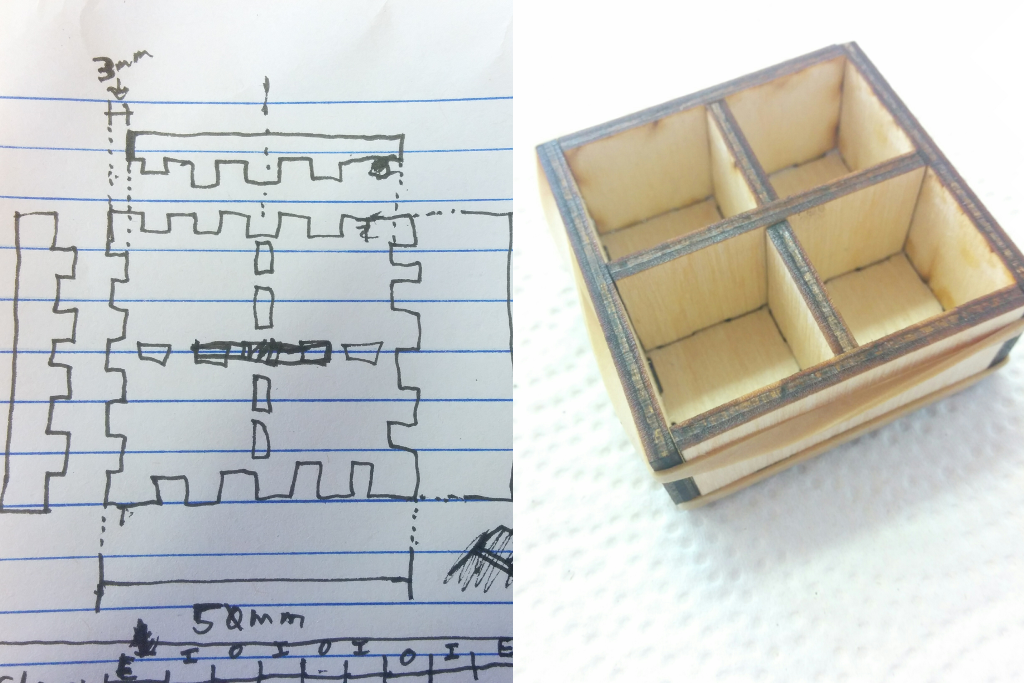
Quick sketch of a test box, and what it’ll look like.